This article shows how QA teams and developers may create AI assistants by integrating OpenAI’s cutting-edge AI technologies. In this instance, we are setting up the OpenAI package using our specific API key. This key is essential because it provides us with access to the OpenAI platform and allows us to take advantage of all its features.
Steps to Build the Model and Web App:
Pre-requisite:
Ø Go through this: https://platform.openai.com/docs/quickstart?context=python
Ø Install Python and other dependencies like streamlit, openAI, etc. on your machine using the PIP package installer if you are planning to run it on your local.
Ø Create an Open API account, and generate API key using https://platform.openai.com/api-keys (Note* You receive free $5 when signing up using your mobile phone, which is sufficient for you to play with.)
Step 1: Create a GitHub Account and Create a New Repository
- Go to GitHub’s website.
- Click on the “Sign up” button in the top-right corner. Provide valid details to sign-up.
- Once logged in, click on the “+” icon in the top-right corner and select “New repository.”
- Give your repository a name, such as “test-case-review”
- Choose if you want your repository to be public or private.
- Initialize the repository with a README file.
- Click “Create repository.”
Step 2: Add Files to Your Repository
- In your repository, click on “Add file” and select “Create new file.”
- Create a file named
app.py
—this will be your main Python file for the Streamlit app. - Write your Streamlit code into
app.py
. - Create another file named
requirements.txt
. This file should list all Python libraries that your app depends on, includingstreamlit
andopenai
. - Commit the new files by clicking “Commit new file.”
Here is app.py:
import os
import openai
import streamlit as st
import time
# Retrieve the API key from the environment variable
OPENAI_API_KEY = os.getenv('OPENAI_API_KEY')
# Initialize the OpenAI client with the API key
openai.api_key = OPENAI_API_KEY
# Create the Assistant
try:
assistant = openai.beta.assistants.create(
name="Test Automation Co-Pilot",
instructions="You are an AI assistant specialized in software testing. Analyze the provided test scripts/use cases and offer solutions and optimizations based on them.",
tools=[{"type": "retrieval"}],
model="gpt-3.5-turbo"
)
except Exception as e:
st.error(f"An error occurred while creating the Assistant: {e}")
raise
# Streamlit components
st.title("Test Cases Optimization Assistant")
user_query = st.text_area("Enter your test cases/use cases here to review and hit CTRL+Enter to start processing:", height=150)
if user_query:
try:
thread = openai.beta.threads.create()
message = openai.beta.threads.messages.create(
thread_id=thread.id, role="user", content=user_query
)
run = openai.beta.threads.runs.create(
thread_id=thread.id,
assistant_id=assistant.id,
instructions="Analyze and suggest optimizations based on the test scripts.",
)
with st.spinner("Processing your request to review the test cases/use cases that you entered above..."):
while True:
run = openai.beta.threads.runs.retrieve(
thread_id=thread.id, run_id=run.id
)
if run.completed_at is not None:
break
time.sleep(7)
messages = openai.beta.threads.messages.list(thread_id=thread.id)
st.header("Assistant's Suggestions:")
for message in messages.data:
if message.content and message.content[0].text.value.strip() and message.role=="assistant":
content = message.content[0].text.value
else:
content = ""
st.write(content)
except Exception as e:
st.error(f"An error occurred while processing your request: {e}")
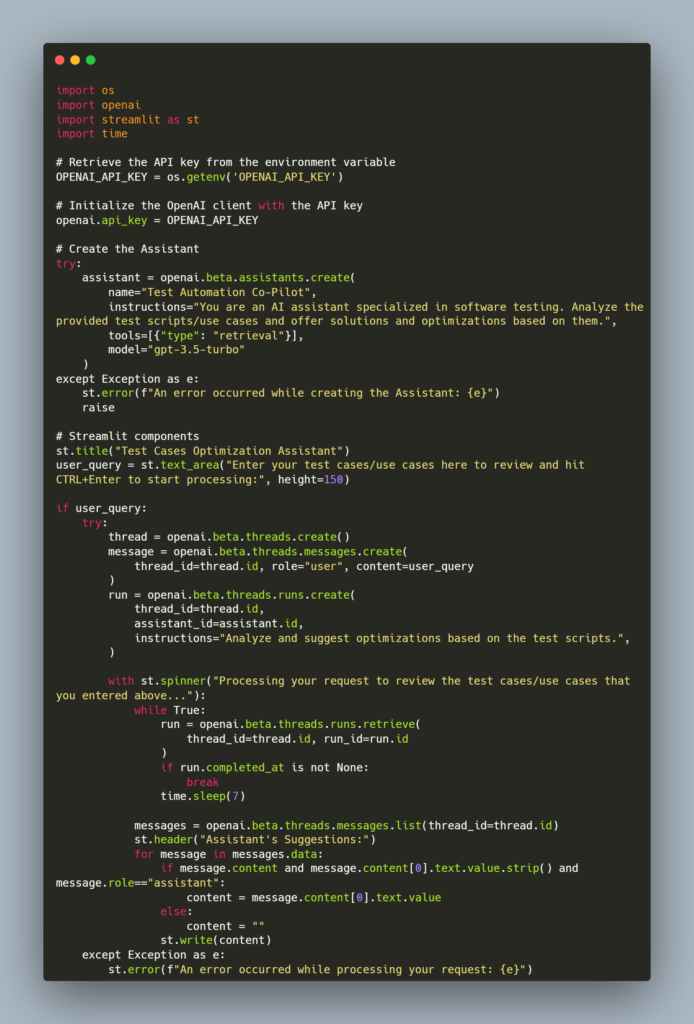
Here is requirements.txt:
streamlit
openai
Step 3: Add Your API Key as a Secret
- Go to your GitHub repository’s Settings tab.
- Find the “Secrets” section in the left sidebar and click on “Actions.”
- Click on “New repository secret.”
- Name your secret (e.g.,
OPENAI_API_KEY
) and paste your OpenAI API key as the value. Note* This is the key that you created from https://platform.openai.com/api-keys. - Click “Add secret” to save it.
Step 4: Create a Share.streamlit.com Account
Visit https://share.streamlit.io/ and click on “Connect GitHub account” to sign up and link your Streamlit account with GitHub.
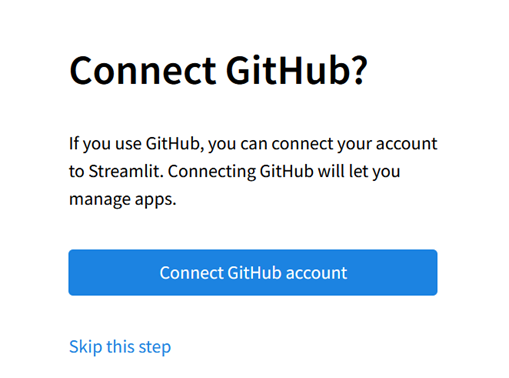
Step 5: Deploy Your Streamlit App
- Once logged into Streamlit, click on “New app.”

2. Select the GitHub repository you created earlier.

3. Choose the branch where your files are located.

4. Write app.py
in the “Main file path” field.

5. In the “Advanced settings,” input your secret key (OPENAI_API_KEY
) into the “Environment variables” section. P.S.: Ensure that you add the correct API Key.
OpenAI API key usually starts with “sk-“.
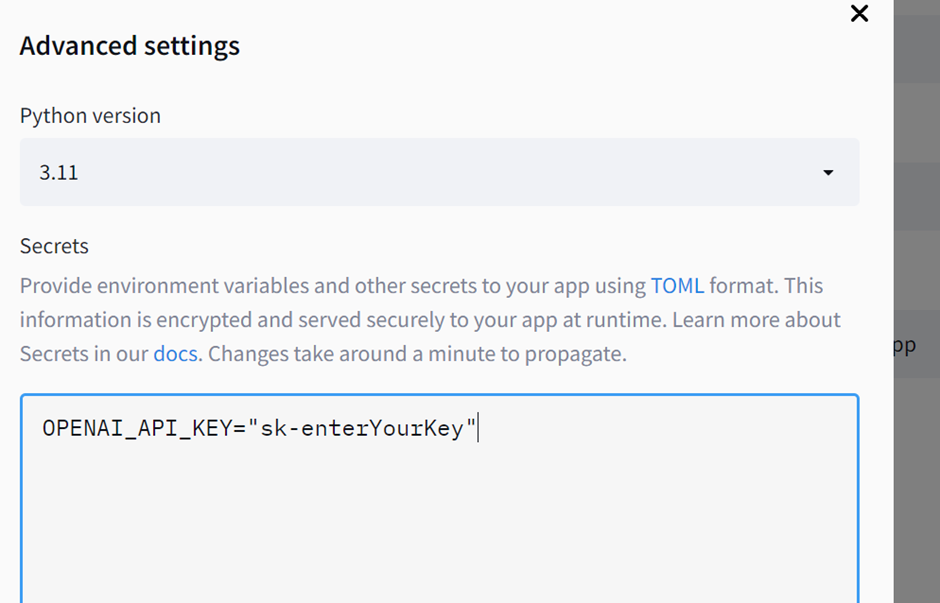
6. Click “Deploy” to deploy your app. Streamlit will automatically install the dependencies listed in your requirements.txt
file and deploy your app.
You are all set:
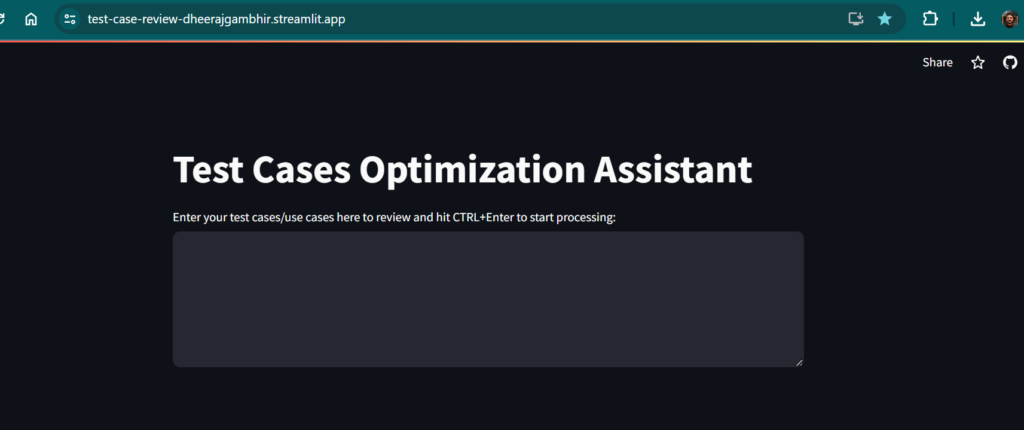
Have posted the execution video here: https://softwaretestingjournal.com/openai-learning-chapter-3/